Background Location Tracking in React Native
A live demo of this application that you can also run on your mobile phones is available at this expo snack. Just download Expo app from Play Store, scan the code and voila! Please update the packages used to latest version before using.
React Native is an amazing JavaScript framework for developing mobile applications. It was developed by Facebook and was open-sourced in 2015. React Native or RN enables us to make platform independent applications, meaning there will be a single codebase for both Android and iOS platforms. It allows developers to use the functionalities and structure of ReactJs with native platform capabilities.
We will use Expo to build native react apps. Expo is a very powerful framework and a platform that supports all universal React Applications. It basically provided us with a set of comprehensive tools and built-in services to develop, build and deploy Android, iOS and web applications with ease from a single JavaScript/TypeScript codebase. For web developers who are wanting to start mobile development or have a project that requires rapid development, expo is the thing they should start with. Expo also provides easy to use predefined libraries for all kinds of purposes. One really interesting library is expo-location which can be used to find user location. We can combine expo-location with expo-task-manager to track the location at regular intervals when the application is running in the background. In this blog, we will see how we can leverage expo-location along with the expo-task-manager to track the user’s location in real time and log it on the console.
So, spin up your react native application and let’s begin!
The first thing we need to do is download some expo packages,
npm install expo-location expo-task-manager
Or if you are using yarn,
yarn add expo-location expo-task-manager
Now, at starting we have a basic react native app like this,
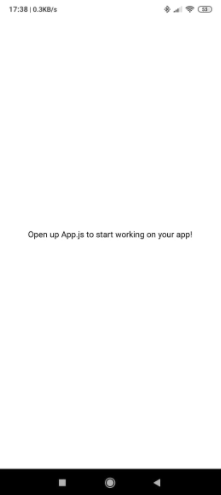
Create a new file named “UserLocation.js”.
Add this piece of code to the UserLocation.js file.
Here we have defined a Task Name, “Location_Tracking” which will start when we press a button, and it will keep logging our real time location on the console. You can also integrate a backend architecture and store the location in some database using APIs. As location parameters, we are provided with latitude, longitude, accuracy, wind speed and many more. We all know what latitude and longitude are, they pinpoint the location of our mobile phone on a map. Accuracy is the precision or the probability percentage representing exactly how trustworthy this location is. Of course, in order to share a location you must allow the application to capture your location. The task of location tracking is defined using expo-task-manager. And it works seamlessly across all component even while navigation, it just keeps running in the background. You can start and stop the running task anytime, which in turn will automatically start or stop tracking your location.
Change the App.js file to render UserLocation component,
import React from 'react';
import { StyleSheet, View } from 'react-native';
import UserLocation from './UserLocation';export default function App() {
return (
<View style={styles.container}>
<UserLocation />
</View>
);
}const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
});
The interface now looks like,

Reload the mobile application and there you have it. Press the “Start Tracking” button and check the console on metro bundler (switch to the console with the model name same as your phone), you will see the latitude and longitude being logged along with present date and time every 10 seconds, you can of course change this time interval according to your needs.
